30 Aug 2019
Browserify Transform Error : ParseError: ‘import’ and ‘export’ may appear only with ‘sourceType: module’
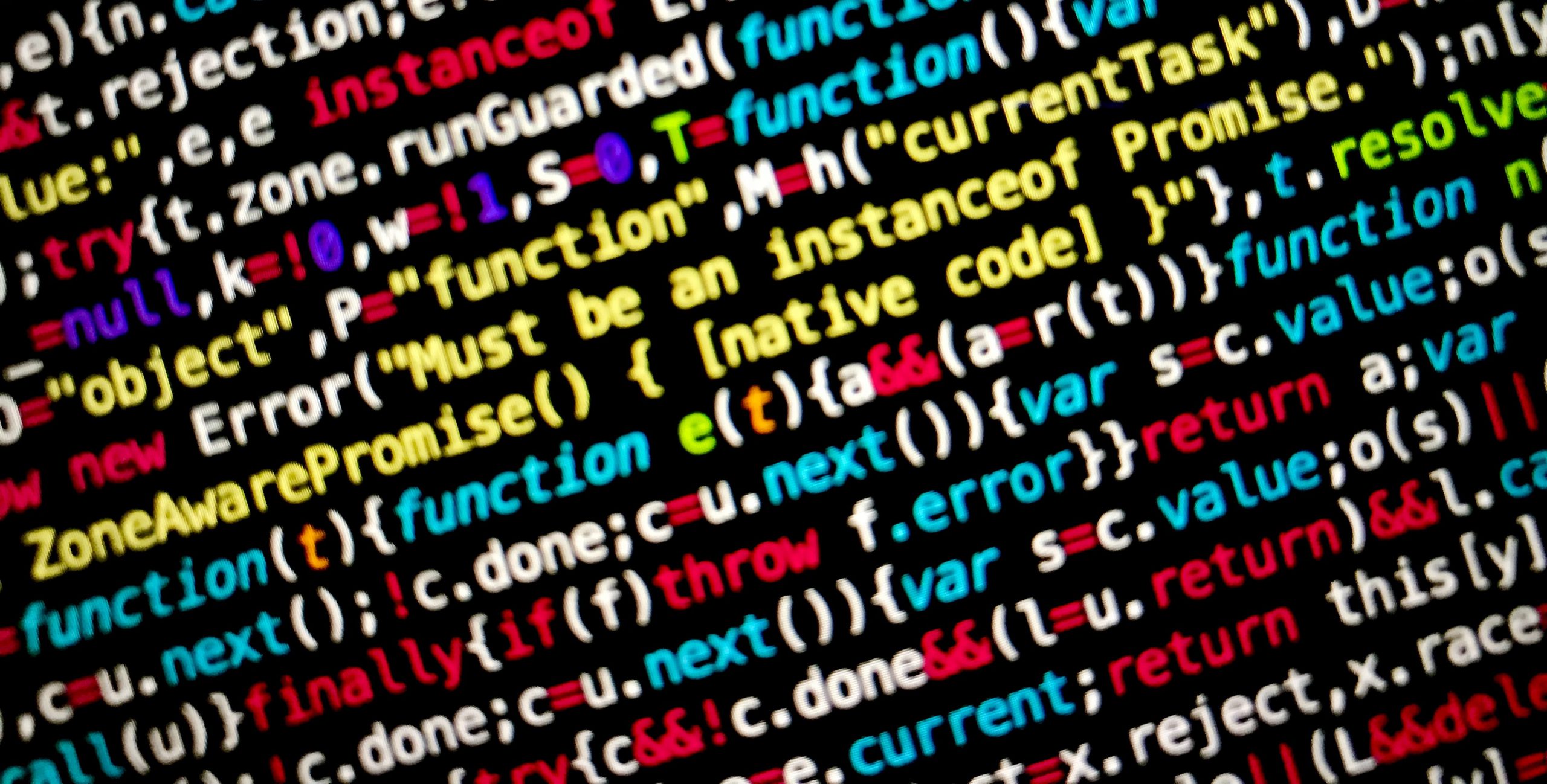
This error hit me when i was trying to use Swiper’s custom build. In my gulp setup I make use of browserify and babelify like this:
gulp.task('js', (done) => {
browserify('./src/js/main.js', {debug:true})
.transform('babelify', {
presets: ['@babel/preset-env']
}) //transpile es6 to es2015
.bundle()
.on('error', gutil.log.bind(gutil, 'Browserify Error'))
.pipe(source('bundle.js'))
.pipe(buffer())
.pipe(sourcemaps.init({loadMaps: true}))
.pipe(mode.development(uglify()))
.pipe(mode.local(uglify()))
.pipe(mode.production(
uglify({
compress: {
drop_console: true
}
})
))
.pipe(sourcemaps.write('./'))
.pipe(gulp.dest('./dist/js'))
done(browserSync.reload())
})
The error after running this task was:
node_modules/swiper/dist/js/swiper.esm.js:13
import { $, addClass, removeClass, hasClass, toggleClass, attr, removeAttr, data, transform, transition as transition$1, on, off, trigger, transitionEnd as transitionEnd$1, outerWidth, outerHeight, offset, css, each, html, text, is, index, eq, append, prepend, next, nextAll, prev, prevAll, parent, parents, closest, find, children, remove, add, styles } from 'dom7/dist/dom7.modular';
^
ParseError: 'import' and 'export' may appear only with 'sourceType: module'
After a pretty long search this is the solution i came up with:
Babelify does not process any node_modules by default if your package is making use of es6 modules just like swipers custom builds, you need to include those dependencies in the gulp file like this:
browserify('./src/js/main.js', {debug:true})
.transform('babelify', {
global: true, //include all of the files in the root
ignore: [/\/node_modules\/(?!swiper|dom7\/)/], //exclude node modules but process swiper and dom7
presets: ['@babel/preset-env']
})
dom7 needs to be included too since swiper is making use of it.